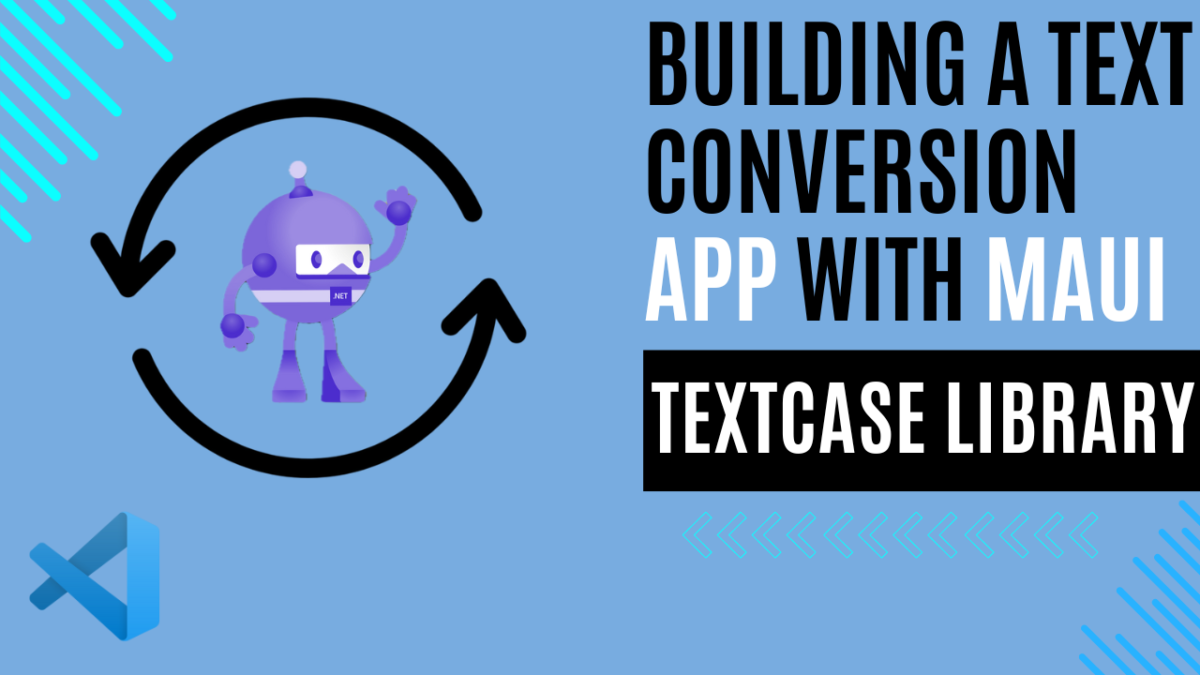
Building a Text Conversion App with MAUI and TextCase Library
Discovering TextCase: Simplifying Text Manipulation
Welcome to TextCase 1.0.11, a project born out of passion and meticulously crafted three years ago. Its mission? To help developers avoid wasting time on mundane text conversions within the .NET ecosystem.
TextCase isn’t just another library; it’s a powerhouse engineered to streamline text transformation tasks. With an array of 19 distinct conversion methods, ranging from the fundamental UpperCase and LowerCase to the intricacies of TitleCase, CamelCase, PascalCase, and even Base64 encoding/decoding, TextCase offers unparalleled versatility.
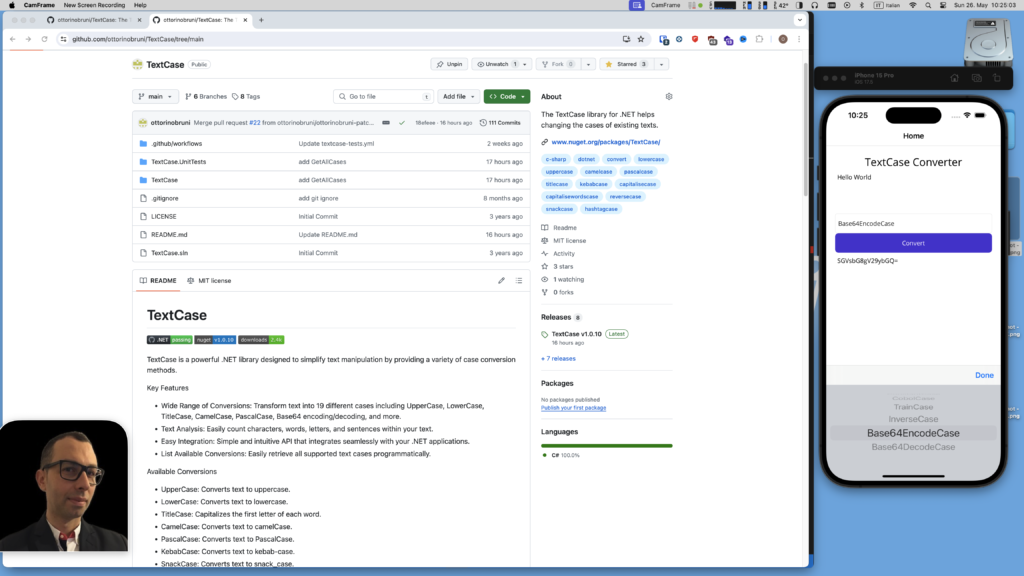
But that’s not all. TextCase goes beyond simple conversion; it empowers developers to analyze text effortlessly. Metrics like character count, word count, letter count, sentence count, and paragraph count are at your fingertips, enabling thorough text assessment with ease.
Integration is seamless, thanks to TextCase’s intuitive API. Whether you’re a seasoned developer or just starting out, embedding TextCase within your .NET applications is a hassle-free experience, eliminating complexity and streamlining your workflow.
Available Conversions
- UpperCase: Converts text to uppercase.
- LowerCase: Converts text to lowercase.
- TitleCase: Capitalizes the first letter of each word.
- CamelCase: Converts text to camelCase.
- PascalCase: Converts text to PascalCase.
- KebabCase: Converts text to kebab-case.
- SnackCase: Converts text to snack_case.
- HashTagCase: Converts text to #HashTagCase.
- ConstantCase: Converts text to CONSTANT_CASE.
- CobolCase: Converts text to COBOL-CASE.
- InverseCase: Alternates case starting with lowercase.
- TrainCase: Converts text to Train-Case.
- CapitaliseCase: Capitalizes the first letter of the text.
- CapitaliseWordsCase: Capitalizes the first letter of each word.
- ReverseCase: Reverses the text.
- AlternateCase: Alternates case starting with uppercase.
- Base64EncodeCase: Encodes text to Base64.
- Base64DecodeCase: Decodes Base64 text.
Text Analysis Examples:
- number of characters
- number of words
- number of letters
- number of sentences
- number of paragraphs
And if you’re interested in contributing, feel free to fork the project your collaboration is more than welcome.
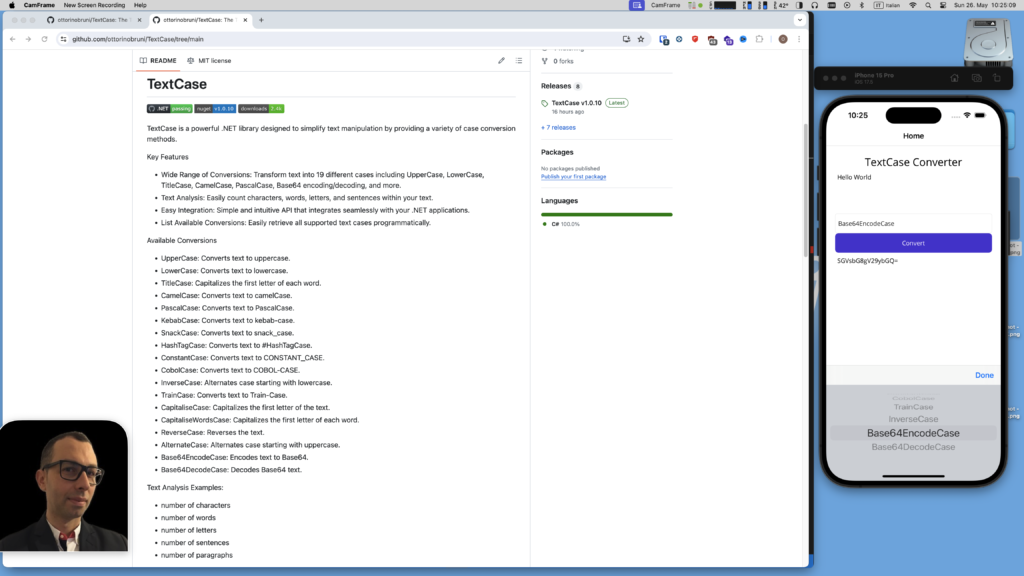
Using TextCase with a .NET MAUI Project
Welcome to the TextCase Converter app built with .NET MAUI! Unlike a simple console application, this app provides a user-friendly interface for effortless text manipulation on iOS, with the potential for expansion to Android, macOS, and Windows platforms.
The app features a clean and intuitive layout, consisting of:
- TextCase Converter Title: A prominent title at the top of the app interface, indicating its purpose.
- Input Text Editor: An input field where users can enter the text they wish to convert.
- Case Selection Picker: A dropdown menu allowing users to select the desired text conversion case.
- Convert Button: A button that triggers the conversion process when clicked.
- Output Text Editor: An output field displaying the converted text based on the selected case.
The conversion process is straightforward and seamless. Users simply input the desired text, select a conversion case from the dropdown menu, and click the “Convert” button. The converted text is then displayed in the output field below.
It’s worth noting that, due to time constraints and for educational purposes, this app does not utilize the MVVM (Model-View-ViewModel) pattern. However, the MVVM pattern is considered essential for creating robust and maintainable apps.
By separating concerns and decoupling the user interface from the business logic, the MVVM pattern offers several advantages, including improved code organization, easier unit testing, and enhanced maintainability. In future iterations of this app or similar projects, incorporating the MVVM pattern would be highly beneficial.
This app showcases the ease of integrating the TextCase library into a real-world .NET MAUI project, providing developers with a powerful tool for text manipulation tasks. With its versatile capabilities and platform flexibility, TextCase Converter simplifies text transformations across various .NET-supported platforms.
For more detailed information on developing .NET MAUI apps using Visual Studio Code, refer to my previous article. Happy text converting!
Step 1: Setting Up Your MAUI Project
Begin by creating a new .NET MAUI project in Visual Studio or your preferred IDE. Choose the appropriate project template based on your target platform (e.g., Android, iOS, Windows), and ensure that your development environment is properly configured.
Step 2: Installing TextCase via NuGet
Once your MAUI project is set up, the next step is to install the TextCase library via NuGet. Open your project’s solution in Visual Studio and navigate to the NuGet Package Manager. Search for “TextCase” and install the latest version of the package.
dotnet add package TextCase
Step 3: Implementing TextCase in Your Project
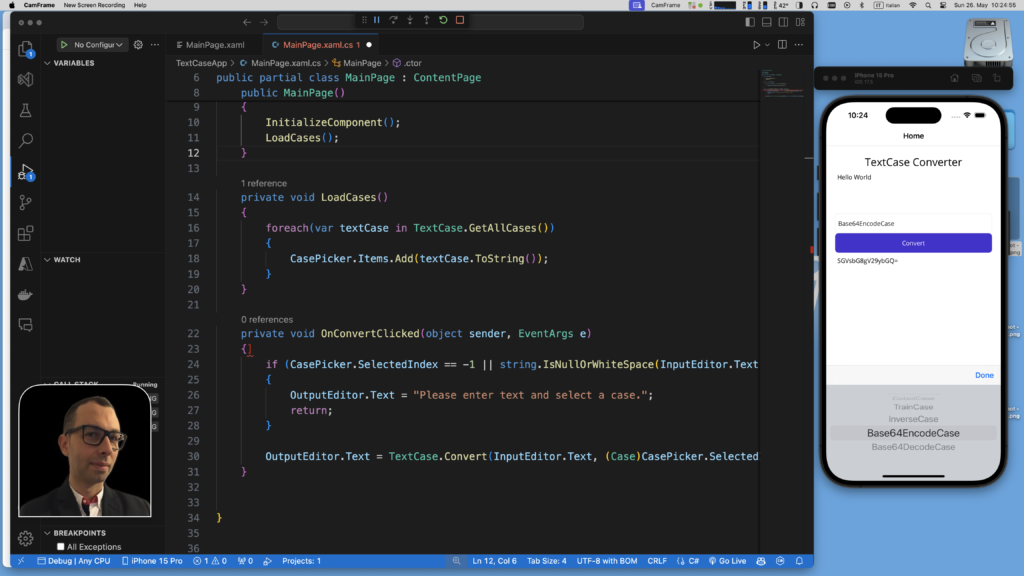
With TextCase installed, you’re ready to incorporate it into your MAUI application. Start by importing the TextCase namespace into your code files:
public partial class MainPage : ContentPage { public MainPage() { InitializeComponent(); LoadCases(); } private void LoadCases() { foreach(var textCase in TextCase.GetAllCases()) { CasePicker.Items.Add(textCase.ToString()); } } private void OnConvertClicked(object sender, EventArgs e) { if (CasePicker.SelectedIndex==-1||string.IsNullOrWhiteSpace(InputEditor.Text)) { OutputEditor.Text="Please enter text and select a case."; return; } OutputEditor.Text=TextCase.Convert(InputEditor.Text, (Case)CasePicker.SelectedIndex); } }
<StackLayoutPadding="20"> <Label Text="TextCase Converter" FontSize="24" HorizontalOptions="Center"/> <Editor x:Name="InputEditor" Placeholder="Enter text here..." HeightRequest="100"/> <Picker x:Name="CasePicker" Title="Select Case"/> <Button Text="Convert" Clicked="OnConvertClicked"/> <Editor x:Name="OutputEditor" Placeholder="Converted text will appear here..." HeightRequest="100" IsReadOnly="True"/> </StackLayout>
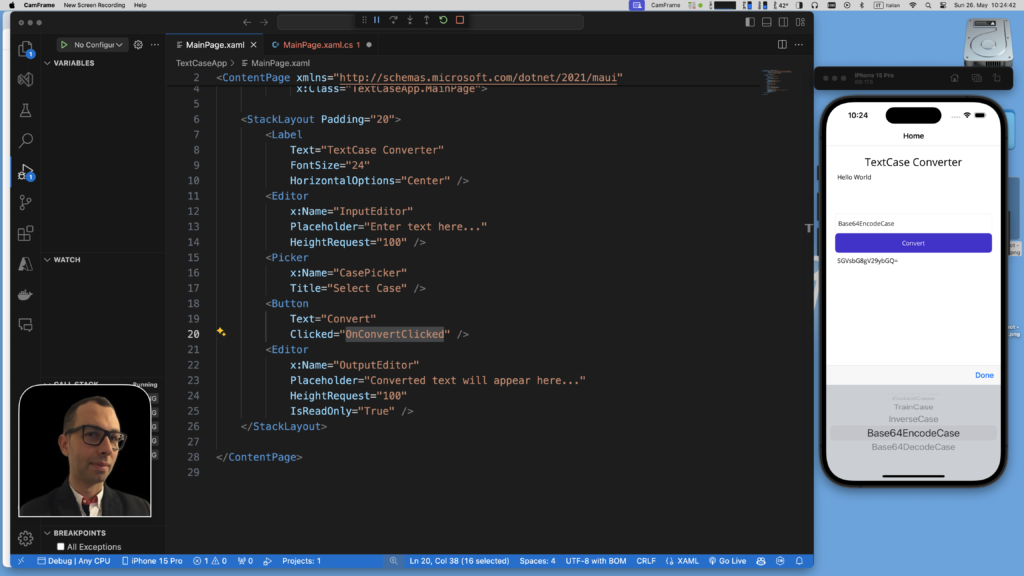
Final Thoughts: TextCase and .NET MAUI Advantages
Have you witnessed the versatility of my TextCase library and the significant time saved through its usage? With its extensive range of functionalities and the convenience of extensions, transforming a string into the desired case format has never been easier. I encourage you to leverage its capabilities in your projects and kindly report any bugs you encounter.
Have you seen how easy it is to make an app with .NET MAUI? It’s amazing! With only a few lines of code, you can create an app that changes text to any case you want. Just compile it, and it works on almost any device.
These points highlight how TextCase and .NET MAUI make development simpler. They help developers get things done quickly and easily. Let’s use these tools to improve our development and explore new ideas in our projects.

If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!