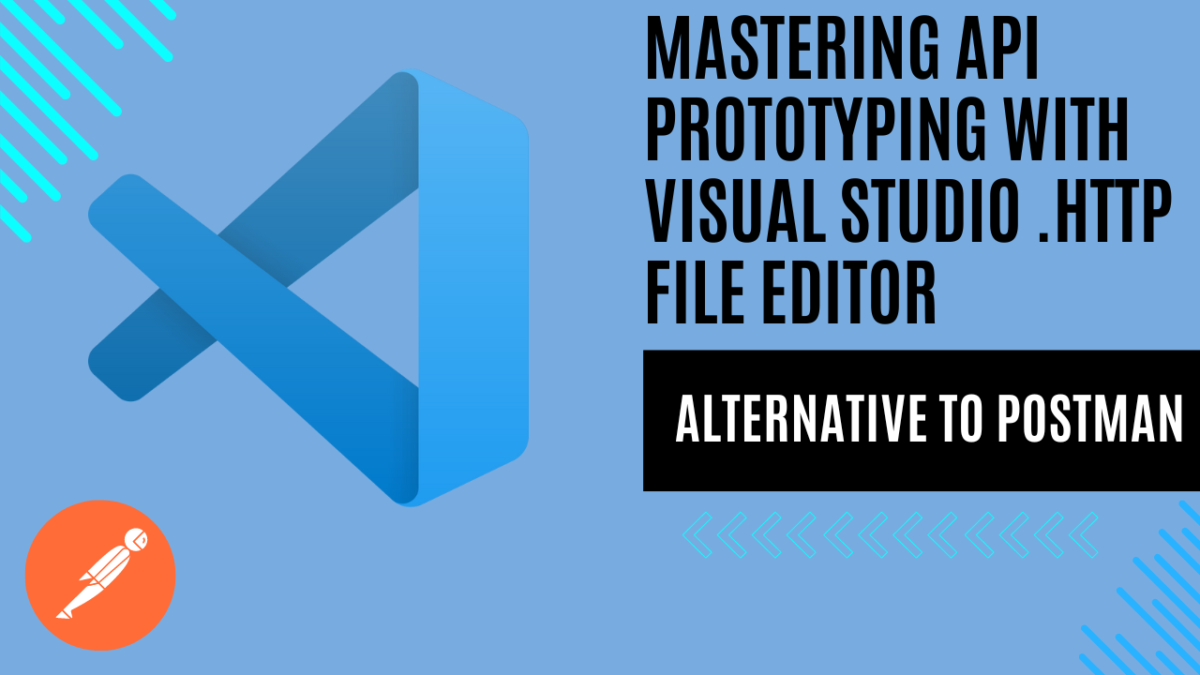
Mastering API Prototyping with Visual Studio .http File Editor: A Cost-Effective Alternative to Postman
Introduction to REST API Development and Validation
Developing a REST API is the most common method for sharing data from a web application. It provides basic CRUD operations to interact with a database, making services more independent. For individual developers, the free plan of Postman works well. However, for simple test calls, upgrading to an enterprise plan can start to become costly. This is where Visual Studio 2022 comes to the rescue.
Exploring the Visual Studio 2022 .http File Editor
The Visual Studio 2022 .http file editor offers a user-friendly solution for testing ASP.NET Core projects, particularly API apps. With its intuitive interface, the editor facilitates:
- Creation and modification of .http files.
- Sending HTTP requests as specified in .http files.
- Displaying responses seamlessly.
The .http
file format and editor was inspired by the Visual Studio Code REST Client extension. The Visual Studio 2022 .http
editor recognizes .rest
as an alternative file extension for the same file format.
Prerequisites Visual Studio 2022 version 17.8 or later with the ASP.NET and web development workload installed.
The format for an HTTP request is HTTPMethod URL HTTPVersion
, all on one line, where:
HTTPMethod
is the HTTP method to use, for example:- OPTIONS
- GET
- HEAD
- POST
- PUT
- PATCH
- DELETE
- TRACE
- CONNECT
URL
is the URL to send the request to. The URL can include query string parameters.HTTPVersion
isHTTP/1.1
,HTTP/2
orHTTP/3
.- The .http syntax is simple:
- `###` to separate different calls definition
- `#` or `//` to comment a line
- `@` to declare a variable
Practical Example: Creating an HTTP File for ASP.NET Core Web API Using Visual Studio
First, I created a Web API project using .NET’s minimal API approach. Below is the code I’ve written.
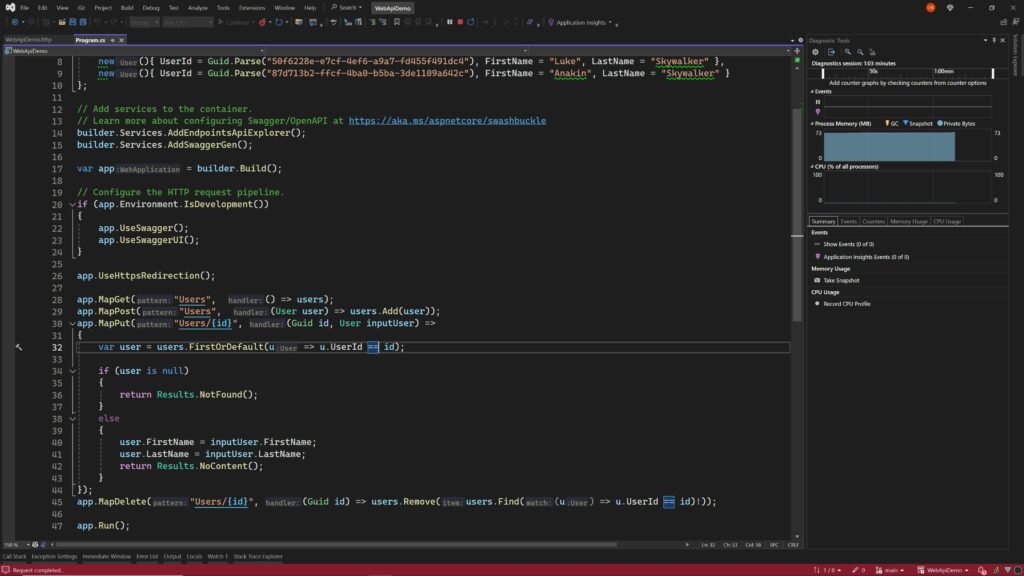
Initially, I defined a basic ‘User’ class within the ‘WebApiDemo’ namespace, containing properties such as ‘UserId’, ‘FirstName’, and ‘LastName’.
public class User { public Guid UserId { get; set; } public string? FirstName { get; set; } public string? LastName { get; set; } }
Next, I implemented the minimal API using the ‘WebApiDemo’ namespace. Here, I instantiated a list of ‘User’ objects to simulate a database. This list contains sample user data.
List<User> users = new() { new(){ UserId = Guid.Parse("c5eb988c-aa12-40ed-838b-6ff34370b8b4"), FirstName="Ottorino", LastName="Bruni" }, new(){ UserId = Guid.Parse("50f6228e-e7cf-4ef6-a9a7-fd455f491dc4"), FirstName="Luke", LastName="Skywalker" }, new(){ UserId = Guid.Parse("87d713b2-ffcf-4ba0-b5ba-3de1109a642c"), FirstName="Anakin", LastName="Skywalker" } };
I’ve defined endpoints for handling CRUD operations related to user data. These endpoints support GET, POST, PUT, and DELETE operations for managing users. This setup serves as a foundation for our API testing and further exploration.
app.MapGet("Users", () => users); app.MapPost("Users", (User user) => users.Add(user)); app.MapPut("Users/{id}", (Guid id, UserinputUser) => { var user = users.FirstOrDefault(u => u.UserId == id); if (userisnull) { returnResults.NotFound(); } else { user.FirstName = inputUser.FirstName; user.LastName = inputUser.LastName; returnResults.NoContent(); } }); app.MapDelete("Users/{id}", (Guid id) => users.Remove(users.Find((u) => u.UserId == id)!));
This HTTP file demonstrates CRUD operations for managing user data using the specified API endpoints. The @HostAddress
variable is set to http://localhost:5157
to define the base URL for the requests. The GET request fetches all users, the POST request adds a new user (Master Yoda), the PUT request updates a user (changing their name to Darth Vader), and the DELETE request removes a user (with the specified user ID).
# Host. @HostAddress = http://localhost:5157 # Retrieves all users. GET {{HostAddress}}/users/ Accept: application/json ### # Adds a new user POST {{HostAddress}}/users/ Content-Type: application/json { "userId": "1902c6a2-7549-4eb5-bcb1-29be91d4a74e", "firstName": "Master", "lastName": "Yoda" } ### # Updates an existing user PUT {{HostAddress}}/users/87d713b2-ffcf-4ba0-b5ba-3de1109a642c Content-Type: application/json { "userId": "87d713b2-ffcf-4ba0-b5ba-3de1109a642c", "firstName": "Darth", "lastName": "Vader" } ### # Deletes a user DELETE {{HostAddress}}/users/1902c6a2-7549-4eb5-bcb1-29be91d4a74e
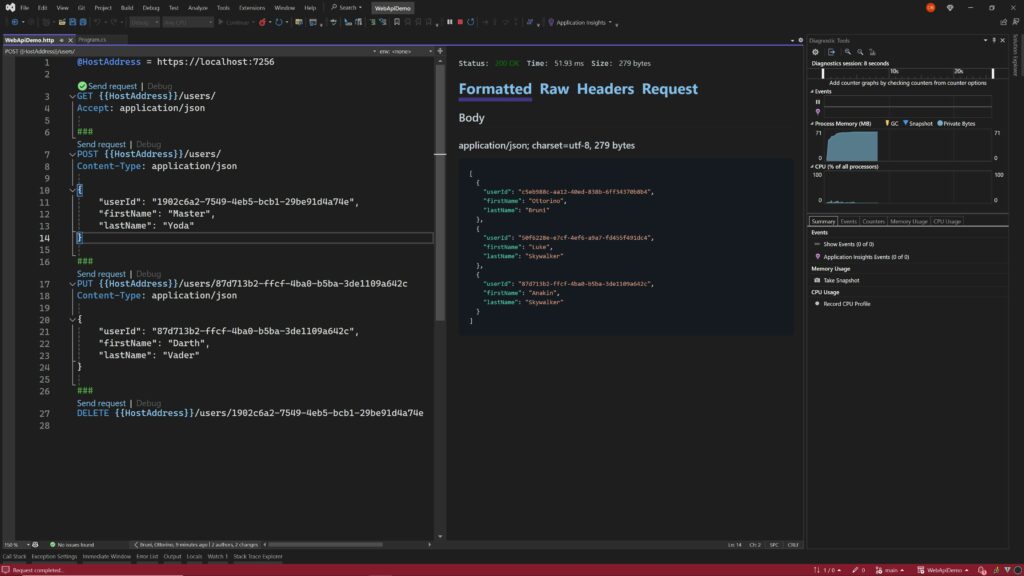
Final Tips and Considerations
In order to manage environment-specific variables effectively, consider creating an http-client.env.json
file. This file should be placed in the same directory as your .http
file or in one of its parent directories.
When working with APIs that require authentication via headers, it’s crucial to avoid storing sensitive information such as secrets or tokens directly in your source code repository. Instead, explore secure methods for managing secrets, such as utilizing ASP.NET Core user secrets, Azure Key Vault, or DPAPI encryption. By adhering to best practices for handling authentication, you can ensure the security of your API integrations and prevent unauthorized access to sensitive data.
Wrap-Up and Conclusions
The integration of the .http file editor in Visual Studio significantly simplifies the process for developers to verify API responses, offering a seamless alternative to external tools like Postman for a wide range of tasks. While the current integration has already enhanced the development workflow, there’s still room for improvement. Future versions of Visual Studio are expected to introduce additional features and enhancements to further enhance the efficiency and effectiveness of API testing within the IDE environment.
Overall, the Visual Studio 2022 .http file editor empowers developers to swiftly send HTTP requests to their Web API endpoints directly from within Visual Studio, providing a more expedient approach to testing endpoints compared to traditional methods such as using Swagger or external tools like Postman.
If you’re interested in exploring the source code of this project, you can find it on GitHub.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!