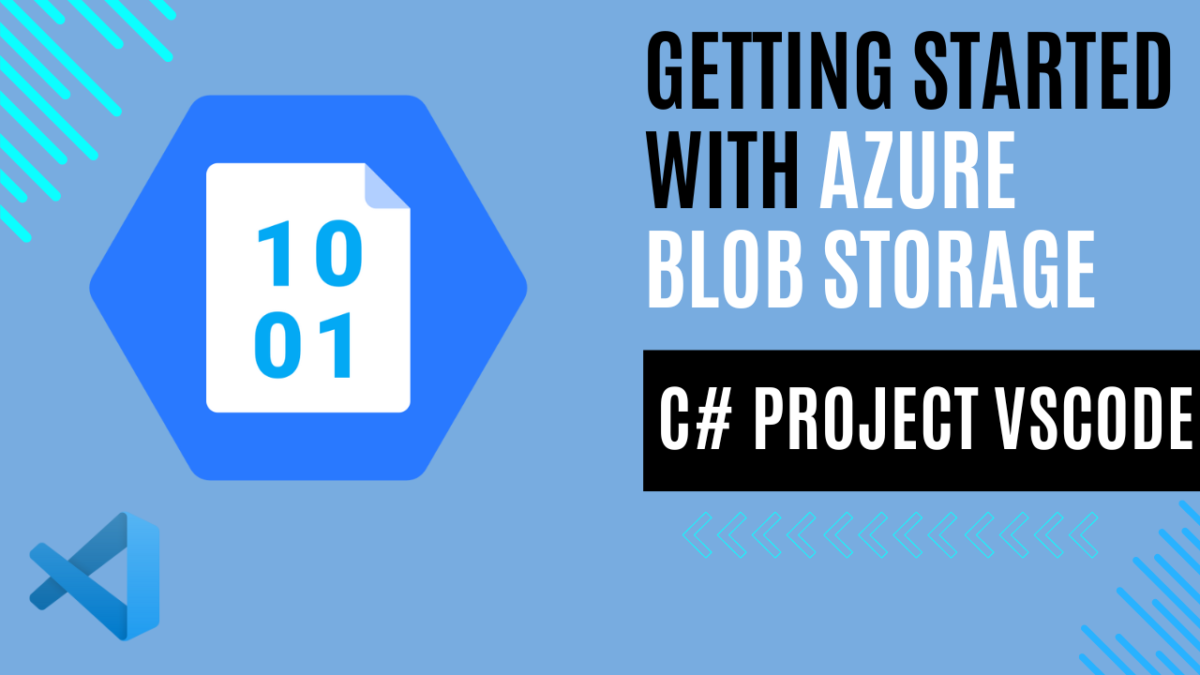
Getting Started with Azure Blob Storage: A C# Project Using VSCode
Introduction
In my recent articles, I’ve talked extensively about Azure Functions. If you haven’t checked them out yet, I recommend starting withGetting Started with Azure Functions: C# and Visual Studio Code Tutorial.
Today, however, I want to talk to you about another very important and powerful Azure service: Azure Blob Storage.
In this article, I won’t delve into architecture or the Microsoft Azure Well-Architected Framework pillars, but I encourage you to explore them if you aim to build efficient, scalable applications. Instead, this tutorial focuses on demonstrating how straightforward it is to leverage Azure Blob Storage with a library.
What is Azure Blob Storage?
Azure Blob Storage is a specialized service within the broader Azure Storage offering from Microsoft. To fully appreciate what Azure Blob Storage is, it’s essential to understand its place within the Azure Storage ecosystem.
Azure Storage
Azure Storage is a cloud storage solution provided by Microsoft Azure that includes various services designed to meet different storage needs. The key components of Azure Storage are:
- Blob Storage: For storing unstructured data like text or binary data.
- Table Storage: For storing structured NoSQL data.
- Queue Storage: For storing and managing message queues.
- File Storage: For shared file storage accessible via the SMB protocol.
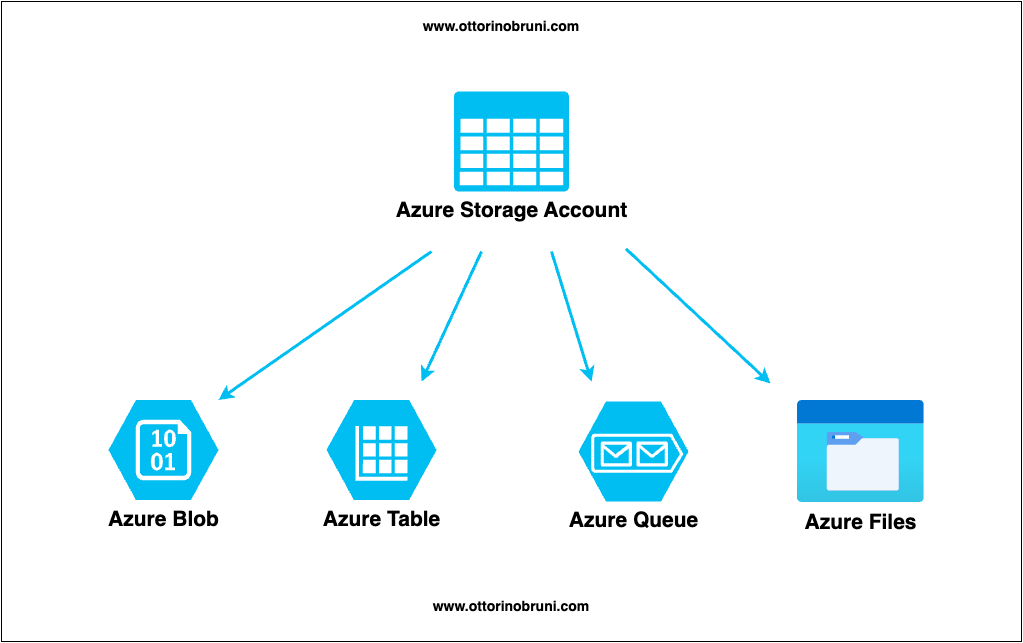
Explore Azure Blob storage
Azure Blob Storage is a specific service within Azure Storage designed to store large amounts of unstructured data. This can include text files, images, videos, backups, and logs, making it highly versatile for various use cases. Here are some of the key features and use cases of Azure Blob Storage:
- Large Data Storage: Ideal for storing large files such as multimedia files, application logs, and backup data.
- Big Data Analytics: Stores data that can be analyzed using on-premises or Azure-hosted services.
- Content Delivery: Serves content such as images, videos, and documents directly to users via web applications.
- Data Backup and Restore: Provides a reliable solution for backing up and restoring data, ensuring high availability and durability.
Azure Storage provides two performance tiers of storage accounts: standard and premium. Each tier offers distinct features and operates on its pricing model.
- Standard: This tier includes the standard general-purpose v2 account, suitable for most Azure Storage use cases.
- Premium: Premium accounts deliver enhanced performance through the utilization of solid-state drives (SSDs). Premium accounts offer flexibility by allowing you to choose between three account types: block blobs, page blobs, or file shares.
Why Use Azure Blob Storage?
- Scalability: Easily scales to meet the needs of applications, whether you’re dealing with a few gigabytes or petabytes of data.
- Accessibility: Accessible from anywhere via HTTP or HTTPS, making it easy to integrate with various applications and frameworks.
- Security: Offers robust security features, including encryption and fine-grained access controls.
Blob Storage Resources
Azure Blob Storage consists of three types of resources:
- Storage Account: This provides a unique namespace in Azure for your data. Every object stored in Azure Storage has an address that includes your unique account name. The account name combined with the Blob Storage endpoint forms the base address for the objects in your storage account.
- Containers: Containers are used to organize sets of blobs, functioning similarly to directories in a file system. A storage account can contain an unlimited number of containers.
- Blobs: Blobs are the actual data objects stored within containers. A container can store an unlimited number of blobs.
The following diagram illustrates the relationship between a storage account, containers, and blobs:
Let’s Create Our Console App in C# and VSCode
It’s time to create our console app in C# using VSCode to demonstrate how easy it is to use Azure Blob Storage.
Prerequisites:
- Azure Subscription: If you don’t have one, you can create an Azure subscription for free.
- Azure Storage Account: You’ll need an Azure Storage account. You can create a storage account if you don’t already have one.
Let’s walk through creating a console app in C# using VSCode to demonstrate how simple it is to work with Azure Blob Storage. We’ll also add a Microsoft library that will make our job easier.
Step 1: Set Up Your Project
- Open VSCode and open a new terminal.
- Create a new project by running the following command:
dotnet new console -o AzureBlobStorageDemo
- Navigate to the project directory:
cd AzureBlobStorageDemo
Step 2: Add the Azure Storage Blobs Client Library
To simplify our interactions with Azure Blob Storage, we’ll use the Azure Storage Blobs client library for .NET.
- Install the package using NuGet by running the following command:
dotnet add package Azure.Storage.Blobs
This library supports multiple server versions and is optimized for storing massive amounts of unstructured data, such as text or binary data.
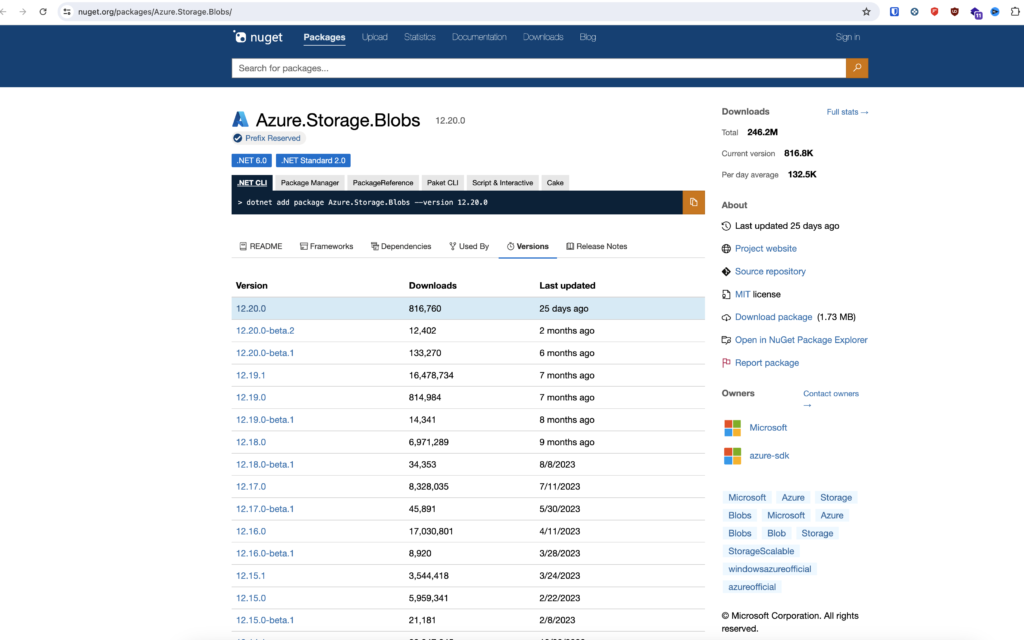
The following packages contain the classes used to work with Blob Storage data resources:
- Azure.Storage.Blobs: Contains the primary classes (client objects) that you can use to operate on the service, containers, and blobs.
- Azure.Storage.Blobs.Specialized: Contains classes that you can use to perform operations specific to a blob type, such as block blobs.
- Azure.Storage.Blobs.Models: All other utility classes, structures, and enumeration types.
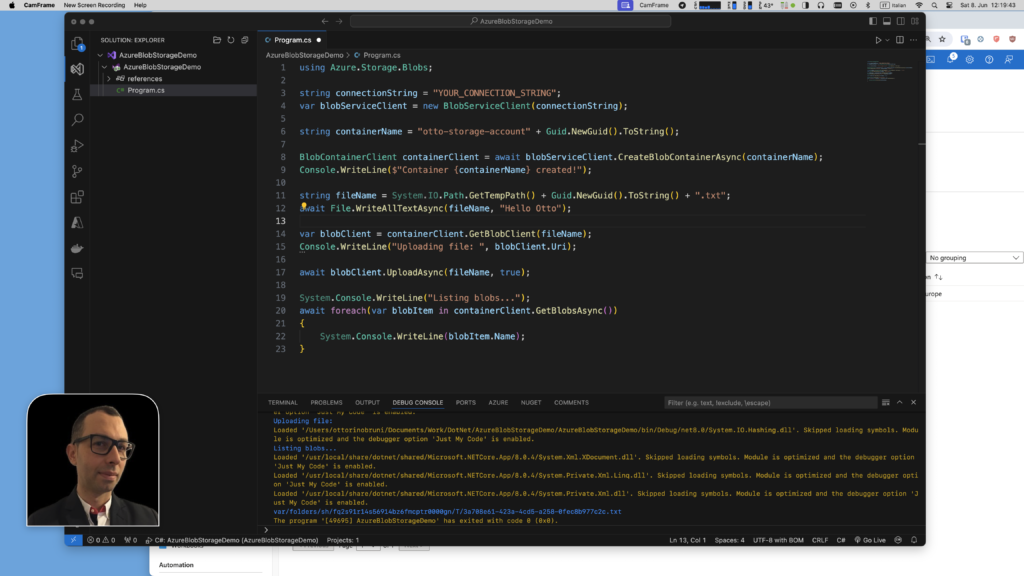
This C# console app, using the Azure.Storage.Blobs
library, demonstrates how to interact with Azure Blob Storage. Here’s a brief description of its functionality:
- Set Up Connection: The app initializes a connection to Azure Blob Storage using a provided connection string.
string connectionString = "YOUR_CONNECTION_STRING";
var blobServiceClient = new BlobServiceClient(connectionString);
- Create a Container: A new blob container is created with a unique name.
string containerName = "otto-storage-account" + Guid.NewGuid().ToString();
BlobContainerClient containerClient = await blobServiceClient.CreateBlobContainerAsync(containerName);
Console.WriteLine($"Container {containerName} created!");
- Create a Temporary File: The app generates a temporary text file with a unique name and writes “Hello Otto” to it.
string fileName = System.IO.Path.GetTempPath() + Guid.NewGuid().ToString() + ".txt";
await File.WriteAllTextAsync(fileName, "Hello Otto");
- Upload the File: The temporary file is uploaded to the newly created blob container.
var blobClient = containerClient.GetBlobClient(fileName);
Console.WriteLine("Uploading file: ", blobClient.Uri);
await blobClient.UploadAsync(fileName, true);
- List Blobs in Container: The app lists all blobs in the container, displaying their names.
System.Console.WriteLine("Listing blobs...");
await foreach (var blobItem in containerClient.GetBlobsAsync())
{
System.Console.WriteLine(blobItem.Name);
}
Finally, you can go to the Azure Portal, navigate to your storage account, and view the newly created container. Inside the container, you will find the text file “Hello Otto” that was uploaded by the app.
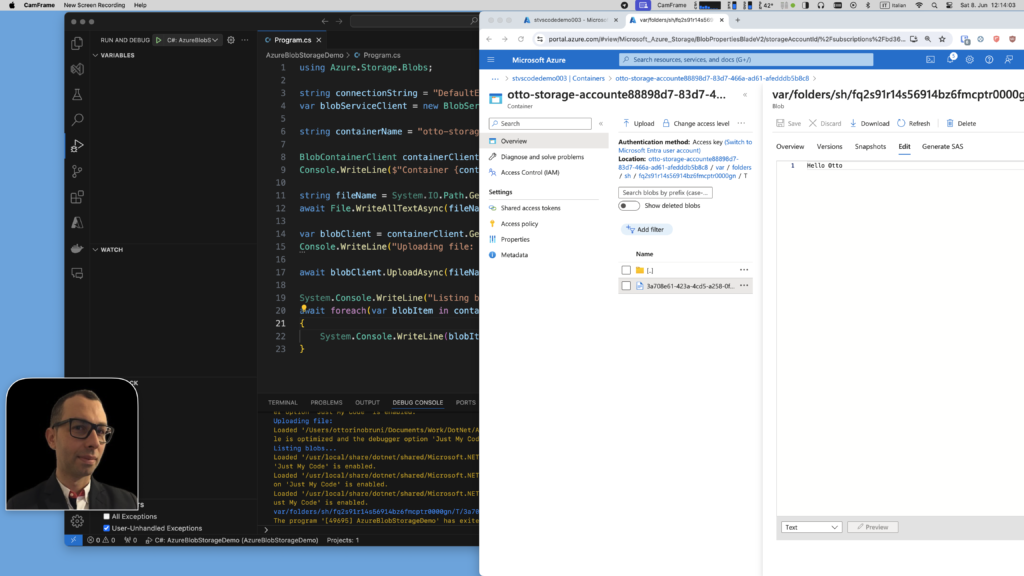
Handling Connection Strings
For this demo, the connection string is included in the code for simplicity. However, for real applications, it’s highly recommended to use environment variables, secrets, or Azure Key Vault to manage the security of your application. You can find the connection string in the Azure portal by navigating to your storage account, then selecting Access keys.
Conclusion
Managing and working with files using Azure Blob Storage and the Azure Storage Blobs client library for .NET is easy and efficient. Azure Blob Storage is powerful and versatile, suitable for many different uses.
Benefits of Using Azure Blob Storage
- Serving Content: Blob Storage is great for showing images or documents directly in a browser.
- Distributed Access: It allows you to store files for access by many users in different places.
- Streaming: You can stream video and audio content easily from Blob Storage.
- Logging: Writing to log files is simple and effective with Blob Storage.
- Backup and Recovery: Blob Storage is excellent for storing data for backup, restore, disaster recovery, and archiving. This keeps your data safe and easy to recover.
- Data Analysis: It supports storing data for analysis by services either on-premises or hosted on Azure.
Using Azure Blob Storage for these tasks shows how flexible and easy it is to use. Whether you need to show content to users, share files, or keep backups, Azure Blob Storage is a reliable and scalable solution.
By using the Azure Storage Blobs client library in your projects, you can manage your data easily, taking full advantage of what Azure Blob Storage offers. This makes it a great choice for many applications, showing its strength and flexibility in handling different types of data in the cloud.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!